The community is working on translating this tutorial into Uzbek, but it seems that no one has started the translation process for this article yet. If you can help us, then please click "More info".
Introduction: Window, DOM & BOM
In the first section of this tutorial, we used quite a bit of time and effort to introduce you to all the general concepts of JavaScript. As we already explained, JavaScript is a programming language used a lot for websites, but it can be used outside of the web as well.
That's why the first section of our tutorial focused 100% on the core concepts of the programming language known as JavaScript, while staying away from the web-specific parts. However, in this next section of our tutorial, this focus will switch completely. We will accept the fact that JavaScript is used by a LOT of developers to make websites more interactive and user-friendly and take you through all the important concepts you need to know about if you want to use JavaScript for the web.
So, if you're all new to this tutorial, and to JavaScript as well, I suggest that you move all the way back to the first section and educate your self on the core concepts of JavaScript, before moving on to this section: When you understand how JavaScript works as a programming language, you are ready to move on to the web-specific parts that we'll cover in this section of the tutorial.
The host environment
While JavaScript was initially created for the web, its now used across all types of devices and platforms - from servers to smart fridges and ovens. JavaScript is free for everyone to implement on their device, to allow developers to write code for it in a well-known, popular and standardized language.
When JavaScript is implemented, you can of course use all the objects and functions already mentioned in the first part of this tutorial, since this is the core-implementation. But a device or application implementing JavaScript can also expose platform-specific functionality, often referred to as the host environment.
So for instance, if a smart oven would implement JavaScript, it would likely expose functionality for turning the oven on and off, regulating the temperature and so on.
When working with JavaScript for web, the browser acts as the host environment and it exposes a lot of extra functionality which allows you, the developer, to interact with the contents of the web page as well as the browser it self. There are many webbrowsers out there, and in the early days of the world wide web, they each had their own, specific implementation of JavaScript, which meant that you couldn't really count on everything working the same way across all browsers. Fortunately, this has become a LOT better over the years and today you can mostly expect things to work the same way across the most popular browsers.
So, when working with JavaScript through a webbrowser, there are some important concepts you need to know about, referred to as the DOM and the BOM. We'll do a brief introduction to them here and then dig much deeper into them later on, but to get started, let's talk about the Window object.
The Window
When a webbrowser acts as the host environment for JavaScript, the top of the object hierarchy is the Window object. Think of the Window object as an object representation of the entire window of your webbrowser, because that's basically what it is. Through this object you can interact with the contents of the website, but also the browser it self.
Here's a diagram I created to illustrate the hierarchy of functionality in JavaScript:
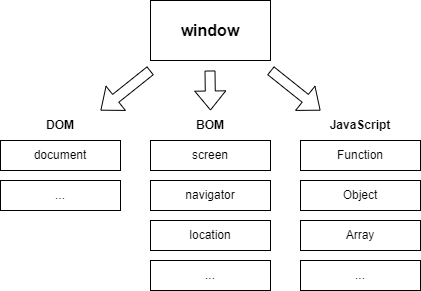
As you can see, it all starts with the window object, which we'll talk much more about in an upcoming article. Below that is the DOM, the BOM and then we have all the core JavaScript functionality already discussed in this tutorial, like functions, objects, arrays and so on.
The Window object comes with lots of functionality. We have already used a bit of it in this tutorial, to illustrate JavaScript functionality. For instance, the alert() method exists on the Window object and allows you to show a popup message in the browser:
window.alert("Hello, window!");
Actually, you can use this function without prefixing it with "window.", because window is the global object in the host environment of a browser. More about this in the dedicated window article later on.
The DOM
Below the window object, you'll find the DOM, short for Document Object Model. It allows you to interact with the contents of a web page through objects. As soon as you start working with JavaScript in a webbrowser, you'll realize that the DOM is VERY important and constantly used. An example of using the DOM could look like this:
alert(window.document.title);
Here, I access the document object on the window and then alert the title of the current page (as declared in the TITLE tag of the page). There's MUCH more to the document object - in fact, I'll dedicate several articles to it later in this tutorial.
The BOM
The BOM, short for Browser Object Model, gives you access to several browser specific objects, allowing you to manipulate certain aspects of the browser (acting as the host environment). For instance, you can access the current URL of the page from the location object:
window.alert(window.location.href);
There are many more objects available from the BOM, so I'll dedicate an entire chapter to this subject later in this tutorial.
Summary
JavaScript was initially created for the web, but is used much wider today. When working with JavaScript for the web, the browser act as the host environment, providing you with added functionality on top of the core JavaScript functionality.
When the browser is acting as a JavaScript hos environment, the window object is the global object, which gives you access to the DOM (Document Object Model) and the BOM (Browser Object Model), which provides access to the content of the web page, as well as specific parts of the browser.
In this section of the tutorial, we will focus solely on JavaScript for the webbrowser and dive into all important aspects of it.